Chapter 4
Assembly Language
MIPS architecture
Architecture Design Principles
1.Simplicity favors regularity
•Consistent instruction format
•Same number of operands (two sources and one destination)
•easier to encode and handle in hardware
2.Make the common case fast
•Only simple, commonly used instructions
•Hardware to decode and execute instructions can be simple, small, and fast
•More complex instructions (that are less common) performed using multiple simple instructions
•MIPS is a reduced instruction set computer (RISC), with a small number of simple instructions
•Other architectures, such as Intel’s x86, are complex instruction set computers (CISC)
3.Smaller is faster
•MIPS includes only a small number of registers
4.Good design demands good compromises
Instruction
MIPS assembly code
Addition: add a , b , c
•add: mnemonic indicates operation to perform
•b,c: source operands (on which the operation is performed)
•a: destination operand (to which the result is written)
Subtraction: sub a , b, c
Multiple Instructions: add t,b,c # t = b + c
Operands
-Registers
•MIPS has 32 32-bit registers
•Registers are faster than memory
•MIPS called “32-bit architecture” because it operates on 32-bit data
MIP Register Set
-
$ before name
-
$0 holds constant value 0
-
saved registers, $s0-$s7, used to hold variables
-
temporary registers, $t0 - $t9, used to hold intermediate values during a larger computation
e.g.
a = b+c
MIPS assembly code: # $s0 = a, $s1 = b, $s2 = c
add $s0, $s1, $s2
-Memory
•Memory is large, but slow
•Commonly used variables kept in registers
Word-Addressable Memory
1.Reading Word-Addressable Memory
•load word (lw)
lw $s0, 5($t1)
–add base address ($t1) to the offset (5)
–address = ($t1 + 5)
–$s0 holds the value at address ($t1 + 5)
2.Writing Word-Addressable Memory
• store word (sw)
sw $t4, 0x7($0)
\ # write the value in $t4
# to memory word 7
Byte-Addressable Memory
Each data byte has unique address
32-bit word = 4 bytes, so word address increments by 4
The address of a memory word must now be multiplied by 4 !!!
MIPS is byte-addressed, not word-addressed!!!
Big-Endian & Little-Endian Memory
-Constants(also called immediates)
Machine Language
1’s and 0’s
•3 instruction formats:
–R-Type: register operands
•3 register operands:
–rs, rt: source registers
–rd: destination register
•Other fields:
–op: the operation code or opcode (0 for R-type instructions)
–funct: the function
–shamt: the shift amount for shift instructions, otherwise it’s 0
–I-Type: immediate operand
•3 operands:
–rs, rt: register operands
–imm: 16-bit two’s complement immediate
•Other fields:
–op: the opcode
–Simplicity favors regularity: all instructions have opcode
–Operation is completely determined by opcode
–J-Type: for jumping
•26-bit address operand (addr)
•Used for jump instructions (j)
Power of the Stored Program
Programming
Logical Instructions
-
and(useful for masking bits): 0xF234012F AND 0x000000FF = 0x0000002F
-
or(useful for combining bit fields):0xF2340000 OR 0x000012BC = 0xF23412BC
-
nor:(useful for inverting bits): A NOR $0 = NOT A
(MIP不提供NOT指令)
andi,ori,xori
Shift Instructions
•sll: shift left logical
–Example:** sll $t0, $t1, 5 # $t0 <= $t1 << 5
•srl: shift right logical
–Example: srl $t0, $t1, 5 # $t0 <= $t1 >> 5
•sra: shift right arithmetic
–Example: sra $t0, $t1, 5 # $t0 <= $t1 >>> 5
•sllv: shift left logical variable
–Example: sllv $t0, $t1, $t2 # $t0 <= $t1 << $t2
•srlv: shift right logical variable
–Example: srlv $t0, $t1, $t2 # $t0 <= $t1 >> $t2
•srav: shift right arithmetic variable
–Example: srav $t0, $t1, $t2 # $t0 <= $t1 >>> $t2
Generating Constants
-
16-bit constants
-
32-bit constants (lui + ori)
lui stands for load upper immediate
Multiplication, Division
-
Special registers: lo , hi
-
32x32 multiplication = 64 bit result
–mult $s0, $s1
–Result in {hi, lo}
-
32/32 division = 32 bit quotient,remainder
–div $s0, $s1
–Quotient in lo
–Remainder in hi
-
Moves from lo/hi special registers
–mflo $s2
–mfhi $s3
Branching
•Types of branches:
–Conditional
•branch if equal (beq)
•branch if not equal (bne)
–Unconditional
•jump (j)
•jump register (jr)
•jump and link (jal): is similar to j,but is used by procedures to save a return address
Conditional Branching(beq)
The Branch Not Taken(bne)
Unconditional Branching(j)
Unconditional Branching (jr)
jr is an R-type instruction
If Statement
If/Else Statement
While loops
For loops
Less Than Comparison
slt相当于boolean
Arrays
-
Base address(address of the first element)
-
first step: load base address into a register
address依次向上加4
Accessing Arrays
# array base address = $s0
lui $s0, 0x1234 # 0x1234 in upper half of $S0
ori $s0, $s0, 0x8000 # 0x8000 in lower half of $s0
lw $t1, 0($s0) # $t1 = array[0]
sll $t1, $t1, 1 # $t1 = $t1 * 2
sw $t1, 0($s0) # array[0] = $t1
⬇️
get the address of array[1] (array[0]的地址加4)
⬇️
lw $t1, 4($s0) # $t1 = array[1]
sll $t1, $t1, 1 # $t1 = $t1 * 2
sw $t1, 4($s0) # array[1] = $t1
Arrays using For Loops
// C Code
int array[1000];
int i;
for (i=0; i < 1000; i = i + 1)
array[i] = array[i] * 8;
#MIP
# initialization code
lui $s0, 0x23B8 # $s0 = 0x23B80000
ori $s0, $s0, 0xF000 # $s0 = 0x23B8F000
addi $s1, $0, 0 # i = 0
addi $t2, $0, 1000 # $t2 = 1000
loop:
slt $t0, $s1, $t2 # i < 1000?
beq $t0, $0, done # if not then done
sll $t0, $s1, 2 # $t0 = i * 4 (byte offset)
add $t0, $t0, $s0 # address of array[i]
lw $t1, 0($t0) # $t1 = array[i]
sll $t1, $t1, 3 # $t1 = array[i] * 8
sw $t1, 0($t0) # array[i] = array[i] * 8
addi $s1, $s1, 1 # i = i + 1
j loop # repeat
done:
Using lb and sb to Access A Character Array
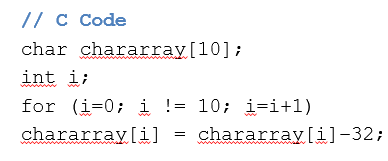
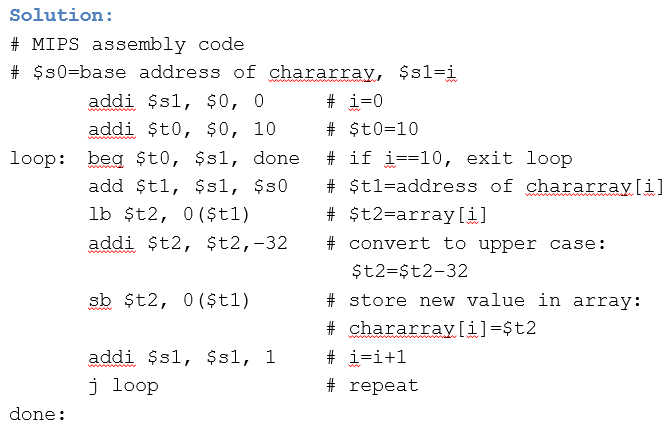
Function calls
-
Caller: calling function
–passes arguments to callee
–jumps to callee
-
Callee: called function
–performs the function
–returns result to caller
–returns to point of call
–must not overwrite registers or memory needed by caller
Stack
•last-in-first-out (LIFO) queue
•Expands uses more memory when more space needed
•Contracts uses less memory when the space is no longer needed
stack pointer($sp):points to top of the stack
Addressing Modes
how to address operands
-
Register Only
Operands found in registers
–Example: add $s0, $t2, $t3
–Example: sub $t8, $s1, $0
-
Immediate
•16-bit immediate used as an operand
–Example: addi $s4, $t5, -73
Example: ori $t3, $t7, 0xFF
-
Base Addressing
base address + sign-extended immediate
–Example: lw $s4, 72($0)
•address = $0 + 72
–Example: sw $t2, -25($t1)
•address = $t1 - 25
-
PC-Relative Addressing(branch and jump)
e.g.
-
Pseudo Direct Addressing
How to Compile & Run a Program
MIPS Memory Map
Odds and Ends
•Pseudoinstructions(伪指令)
•Exceptions
•Signed and unsigned instructions
•Floating-point instructions(浮点指令)